time-based-key-value-store
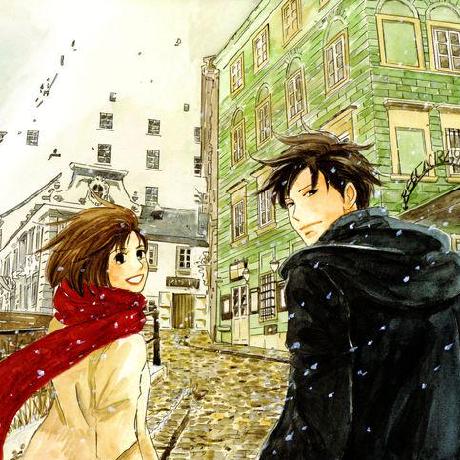
创建一个基于时间的键值存储类 TimeMap,它支持下面两个操作:
- set(string key, string value, int timestamp)
存储键 key、值 value,以及给定的时间戳 timestamp。
- get(string key, int timestamp)
返回先前调用 set(key, value, timestamp_prev) 所存储的值,其中 timestamp_prev <= timestamp。
如果有多个这样的值,则返回对应最大的 timestamp_prev 的那个值。
如果没有值,则返回空字符串(””)。
示例 1:
1 | 输入:inputs = ["TimeMap","set","get","get","set","get","get"], inputs = [[],["foo","bar",1],["foo",1],["foo",3],["foo","bar2",4],["foo",4],["foo",5]] |
示例 2:
1 | 输入:inputs = ["TimeMap","set","set","get","get","get","get","get"], inputs = [[],["love","high",10],["love","low",20],["love",5],["love",10],["love",15],["love",20],["love",25]] |
提示:
- 所有的键/值字符串都是小写的。
- 所有的键/值字符串长度都在 [1, 100] 范围内。
- 所有 TimeMap.set 操作中的时间戳 timestamps 都是严格递增的。
- 1 <= timestamp <= 10^7
- TimeMap.set 和 TimeMap.get 函数在每个测试用例中将(组合)调用总计 120000 次。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/problems/time-based-key-value-store
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
Code
1 | class TimeMap { |
More Info
1. Pair<int,string>
2. Vector<Pair<int,string>>
- 本文标题:time-based-key-value-store
- 本文作者:codeflysafe
- 创建时间:2021-07-10 09:47:01
- 本文链接:https://codeflysafe.github.io/2021/07/10/time-based-key-value-store/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!
评论